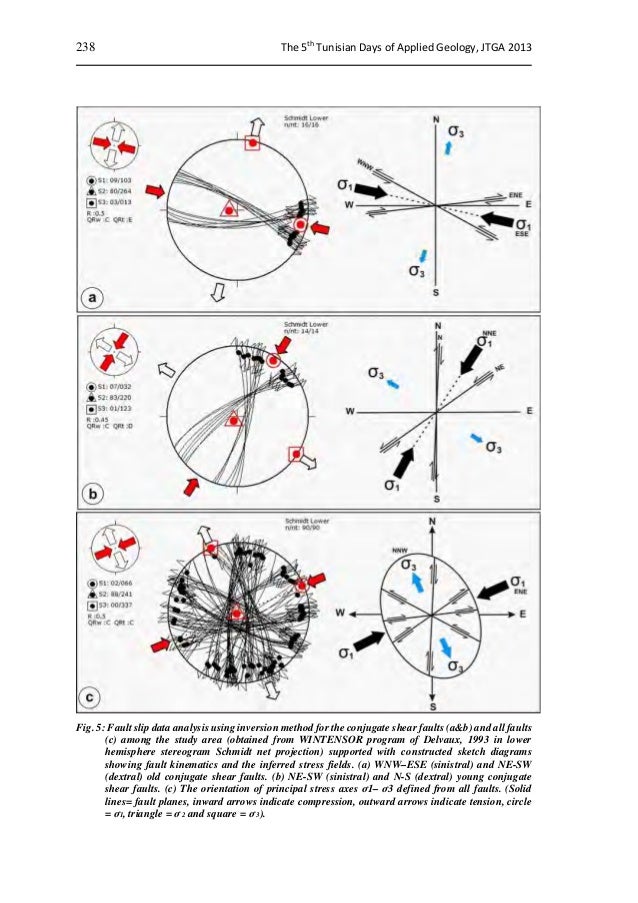
Parallel Projection Program In C
Parallel Projection - Wikipedia. In your image, x and wx is the same axis, and angle is in yoz plane. Projected y: when y = 0, wy = z. cos(pi/2 - α) = z. sin(α) when y 0 and z.
Lab Exercise 5: A Camera for Simple Parallel Projection
This week we construct a module for the simplest kind of projection: orthographicparallel projection. This is the kind of projection used in engineeringdrawings, to produce 'plan views'. The projection is as follows: Givena point (x,y,z) the projection of that point is (x,y). (Alternatively,one could map to (x,z) or (y,z) depending on what kind of plan view isrequired).
Write the following code:
- A simple camera module for managing point coordinates etc
- For rendering to screen
- For performing back face elimination
- Now load 3D objects from file
- Write a program that (a) loads objects from file and then (b) projects them to screen using the simple orthographic projection camera
Your program will be assessed as part of your coursework (10%).
Download the latest version of PES 2013 for Windows. Soccer is back and PES 2013 is ready for this new season. Teams are finishing up the preseason. Pes 2013 pc download full version. Download Pro Evolution Soccer 2013 free offline installer game for all windows 32 and 64 bit. PES 2013 is an simulation sports (soccer) video game by Konami. The game returns to the roots of soccer with unique levels of control plus major emphasis on the individual style. PRO EVOLUTION SOCCER 2013 PC Game is an association football video game in the Pro Evolution Soccer series which abbreviated as PES 2013 and known as World Soccer Winning Eleven 2013 in Japan and South Korea.PES 2011 Pc Game is developed and published by Konami and released on 20 th of September, 2012 in Europe and in North America on 25 September 2012. Pro Evolution Soccer 2013 is the latest version of Konami's popular soccer game for Windows. Although PES 2013 looks very similar to Pro Evoultion Soccer 2012, it includes some subtle changes designed to improved both gameplay and graphics. Download 16,971 downloads. This file will download from PES 2013's developer website. PES 2013 Review. Teams are finishing up the preseason. The signing market, although somewhat affected by the economic crisis, lashes back with trades like that of Jordi Alba to Barcelona or Ibrahimovic to PSG. Meanwhile, in the digital field, PES continues in.
Simple Camera Module
Java Programming
C Programming
Notes on Setting up the ParallelCamera
- The drawable is the physical medium on which the image is rendered, associatedwith the camera. It plays the role of the photographic film in a real camera.
- The boundary defined by [xmin, xmax, ymin, ymax] specifies a 2D windowon the view plane.
- We will take the whole display area as the viewport, hence the viewportcoordinates are (0,0) to (width-1, height -1).
- The values aX, bX, aY, bY are used to convert points on the 2D viewplaneto the 2D display. In other words, if (x,y) is a point on the viewplane,and (x',y') the corresponding point on the display, then x' = aX + bX*x,and y' = aY + bY*y. These values are defined exactly as in the notes on 2D viewing.
- The function clickPCamera() simply computes the values of aX, bX, aY, bYgiven the values of the other parameters.
Rendering
Now we continue with the functions to actually render onto the drawableusing this camera:
Java programming
c programming
Notes
- So the implementation of 'displayFace' is on the following lines:
- Start off a new polygon on the drawable, using 'newPolygonOnDrawable' orusing the drawPolygon method of the Graphics
context for Java programmers; - Suppose (x,y,z) is a vertex of the face, then its projection to the viewplane is (x,y);
- Convert this to display coordinates (x',y') = round(aX+bX*x, aY+bY*y);
- Use the 'addToPolygonOnDrawable' function to send this vertex to the drawingarea;
- When all the vertices of the polygon have been traversed in this way, use'displayPolygonOnDrawable'.
- Problem is that this says nothing about the colour in which to draw theface. This can be easily rectified:
- Add a new entry to the Face data structure, and a corresponding function:
- Before actually drawing the polygon call setColourOnDrawable(face->red,face->green, face->blue).
- To implement 'displayObject', run through all faces in the object calling'displayFace'.
Back Face Elimination
For any polyhedral object obviously not all of its faces can be simultaneouslyvisible from any viewpoint. The kind of parallel projection we have definedis equivalent to looking on a line of sight down the positive Z axis, towardsthe origin (from infinitely far away).
Back face elimination involves displaying only those polygons that arefacing towards the viewpoint. Here is where we can make use of theplane equation of a face. Suppose the plane equation is l(x,y,z) = ax +by + cz -d = 0. Then we know that l(X,Y,Z) > 0 when (X,Y,Z) is 'in frontof' the polygon, ie, the polygon is facing towards (X,Y,Z).
So suppose (0,0,Z) (Z>0) is a point on the Z axis. Then, l(0,0,Z) =cZ - d. We require this to be >0 for a visible face. Ie, cZ - d > 0, andhence d < cZ.
Z is arbitrarily large for a parallel projection (it is at 'infinity').So the requirement d < cZ is equivalent to:
if c > 0 then d can be anything (since d < cZ = +infinity is certainlytrue).
if c < 0 then d must be < -infinity (impossible).
if c = 0 then d must be negative.
Hence the requirement is:
display the face which has plane equation parameters (a,b,c,d) if andonly if c > 0 or (c 0 and d < 0).
Loading objects from file
You now need to write a new constructor for GObject which extendsthe present constructor to read in data from a file according to the specified format below. If you are not sure how to go about doing this, please ask the lab assistant
The format is decribed here for a simple object. We are interested in loading a cube and a pyramid.
Note: colour information has been added, but assuming that you use amonochrome machine only, or only care about displaying as wire frame, thisis not necessary. The colours given are suggested only, use whatever youlike.
Test Program
A sample test program (ShowParallel.java) is available (and at /import/teaching/BSc/CG/lib-java). Please note that there are some small bugs in the code that will need fixing. If you can't fix it, please ask the TAs or your fellow students for advice.
Display a parallel projection of the CUBE AND PYRAMID objects (assuming a plan view lookingdown the positive Z axis, towards the origin - hence (x,y,z) projects to(x,y). Style works 2000 serial number search. Such is the nature of orthographic parallel projection, this will bea rather boring view (you should see a square and an triangle).
To make it more interesting, rotate the cube and pyramid about the Yaxis (transformVerticesOfObject) eg, by 30 degrees(pi/6), clear the window and redisplay. Keep doing this, say 12 times,so that the objects should have rotated a full 360 degrees. Betweeneach rotation, clear the display ('clearDrawable') .Important note: when you rotate the vertices of an object, obviouslythe old plane equations are no longer valid. So the transformation functionsshould take this into account.
A working implementation of test program represents a point of assessmentin the coursework.